-
All Levels
-
32 Weeks
-
MIT Certification
-
Industry Immersion
-
Capstone Projects
Overview
This Python Full Stack Development Course, equipping you with front-end, back-end, and database management skills. Learn to build dynamic web applications using frameworks, APIs, and deployment strategies. Gain hands-on experience and prepare for a successful career in web development.
- Full Stack Developer
- Python Developer
- Web Developer
- Software Engineer
- Backend Developer
- Frontend Developer
- Database Administrator
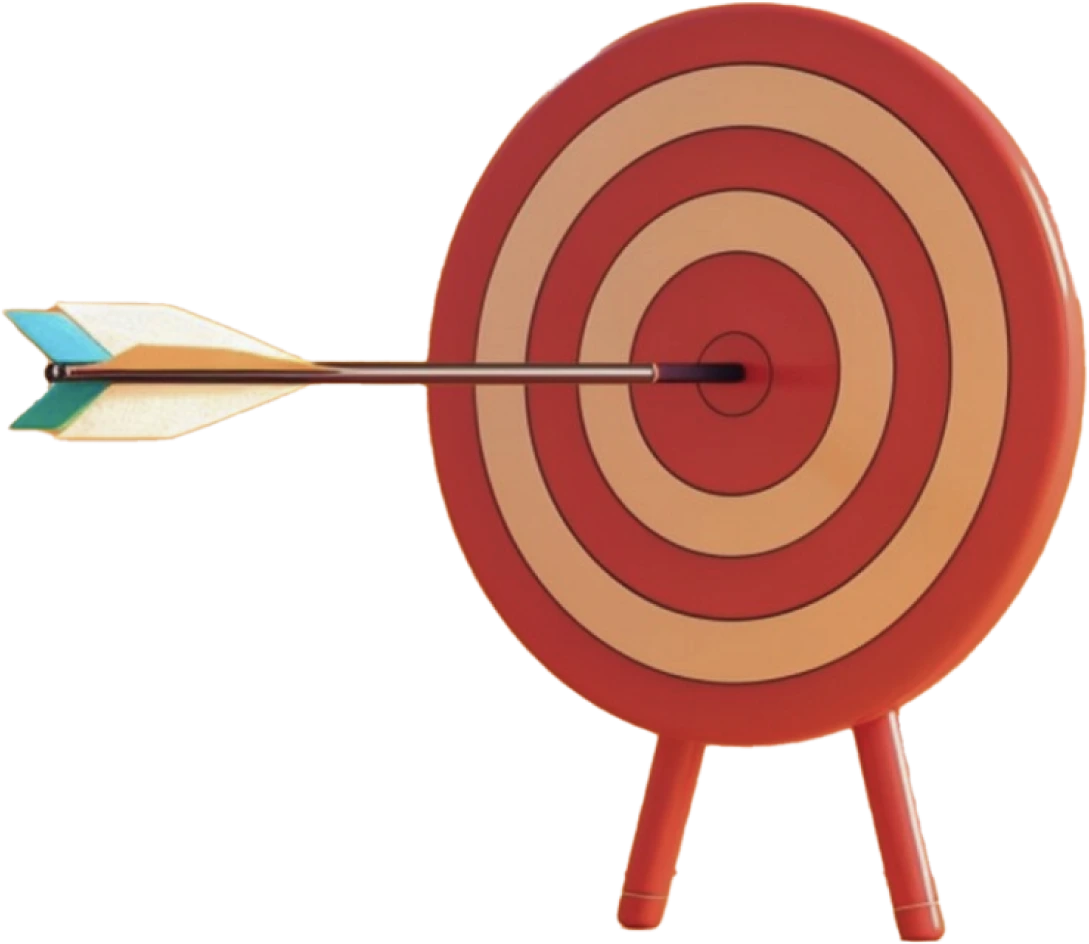
Targeted Job
Roles
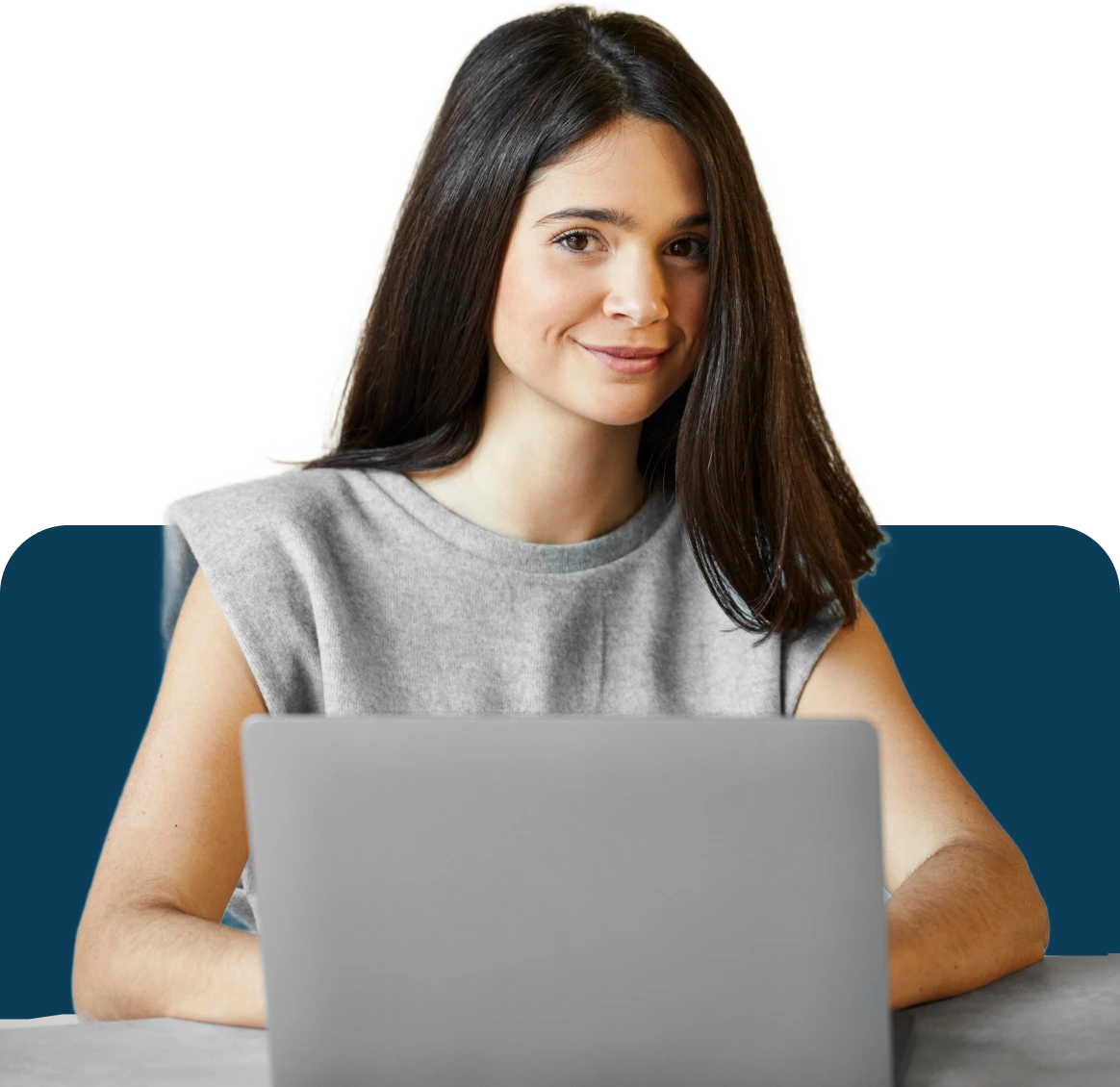
Training and Methodology
By enrolling in this course, you will gain access to -
Hands-On Projects - Build real-world full stack applications using Python.
Front-End Development - Learn HTML, CSS, JavaScript, and frameworks like React.
Back-End Development - Master Python, Django for server-side programming.
Database Management - Learn MongoDB and SQL for efficient data storage and management.
Why Choose This
Course?
Master your skills with Python Full Stack Development Course! Learn front-end (HTML, CSS, JavaScript, React), back-end (Python, Django), and databases (MongoDB, SQL). Build real-world projects, gain hands-on experience, and develop industry-ready skills for a successful career in web development.
Register Now-
100% Job Placement Guarantee
This course guarantees job placement readiness.
-
Real time projects
Apply your skills to industry-relevant challenges in development.
-
Continuous Feedback
Receive regular feedback to enhance your learning journey.
Skills acquired from this course
-
Build responsive UIs with HTML5, CSS3, JavaScript, and React.
-
Develop server-side applications using Python and Django framework.
-
Manage data efficiently with MongoDB and SQL for web applications.
-
Integrate front-end and back-end to create dynamic applications.
-
Work on real-world projects to gain hands-on coding experience.
Tools & Languages Included In This course
The Course Syllabus
The course covers important topics related to Python Full Stack Development.
-
Overview
- Frontend Development
- Backend Development
- Database Management
-
Frontend Development
- HTML5/CSS3
- Introduction to HTML5
- Semantic HTML
- Forms and Input Elements
- Media Elements
- Tables and Lists
- HTML5 APIs and Advanced Features
- CSS3 Basics and Styling
- Attribute Selectors and Styling Basics
- CSS Box Model and Pseudo-classes
- Positioning and Advanced Selectors
- CSS Flexbox and Grid Layout
- CSS Transitions, Animations, and Responsive Design
- Javascript
- Introduction to Javascript
- Operators and Conditionals
- Loops and Iteration
- Functions
- Arrays
- Objects
- DOM Manipulation
- Events
- String Manipulation
- Error Handling
- Fetch API and Promises
- React
- Setting up a Development Environment
- Understanding JSX Syntax
- Creating and Rendering React Components
- Functional vs. Class Components
- Props and State Management in React
- Introduction to useState
- Event Handling in React
- Controlled Components and Handling User Input with Forms
- React Router Basics
- Setting up Routes
- Simple Navigation using Link
- Reusable Components
- Component Lifecycle Methods
- useEffect for Side Effects
- Forms with Validation Techniques
- React Router: Nested Routes, Parameters, and Query Strings
- State Management with Redux
- Actions, Reducers, and Store Setup
- Connecting React Components to Redux
- Asynchronous Actions with Redux Thunk
- Advanced Hooks
- useContext and Creating Custom Hooks
- Performance Optimization
- Advanced React Router
- Fetching and Integrating External APIs in React
- HTML5/CSS3
-
Backend Development
- Python
- Introduction to Python
- Basic Syntax
- Comments and Documentation
- Basic Input and Output
- Data Types and Variables
- Control Flow and Functions
- Error Handling
- Object-Oriented Programming (OOP)
- Classes and Objects
- Inheritance
- Polymorphism
- Encapsulation and Abstraction
- Special Methods
- File Handling
- Advanced Python Concepts
- Decorators
- Generators and Iterators
- Deployment and Version Control
- Introduction to Python
- Django
- Introduction to Frontend
- Introduction to Django
- Displaying Hyperlinks – Project
- Django Architecture
- MVC and MTV
- Creating a Website – Project
- Creating Administration Panel
- Creating First Page of Our Site
- Django Forms Creation
- Django’s Email Functionality
- Django Template Language
- Integrating Bootstrap into Django
- Sessions and Cookies
- Using Other Databases in Django
- Django RESTful API
- Live Project Implementation
- Python
-
Database Management
- MongoDB
- Introduction to MongoDB
- MongoDB Basics
- CRUD Operations
- Basic Querying
- Cursor and Pagination
- Advanced Querying
- Indexing Basics
- Aggregation Framework
- Schema Design
- MongoDB Administration
- MongoDB Tools
- SQL
- Introduction to SQL
- DDL vs DML
- DQL
- Built-in SQL Functions
- Joins and Sub Query
- Stored Procedure and Triggers
- Window Functions
- MongoDB
-
Project Work
Throughout the course, students may be required to complete several projects that demonstrate their understanding and ability to apply the concepts learned. This might include:
- Building a personal portfolio website.
- Developing a web application that incorporates user authentication, data storage, and dynamic content.
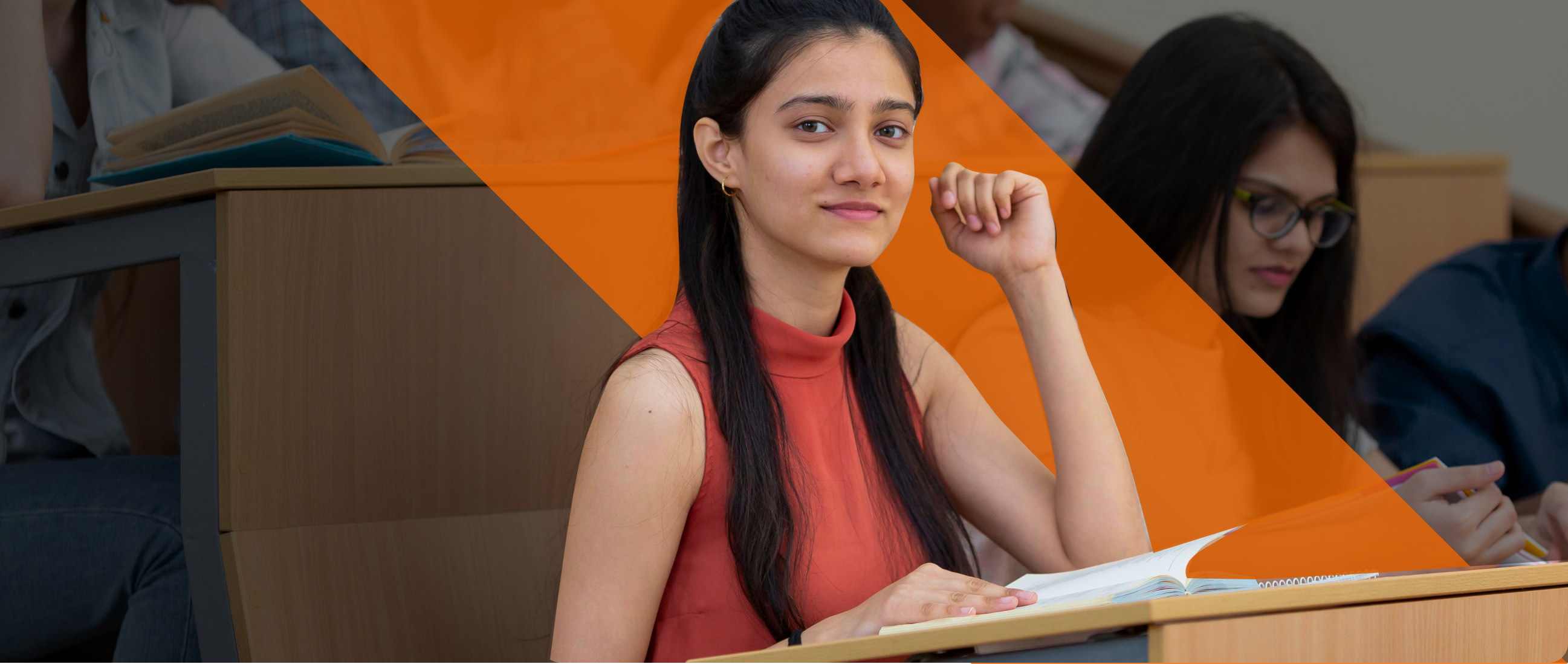
Want to
experience
excellence?
At Milestone, We are committed to provide a
complete education solution in Thane.
Recruiters looking for Full Stack Development Students
Certification For This
Course
Receive a recognized certification upon course completion, validating your skills and boosting your career prospects.
Register Now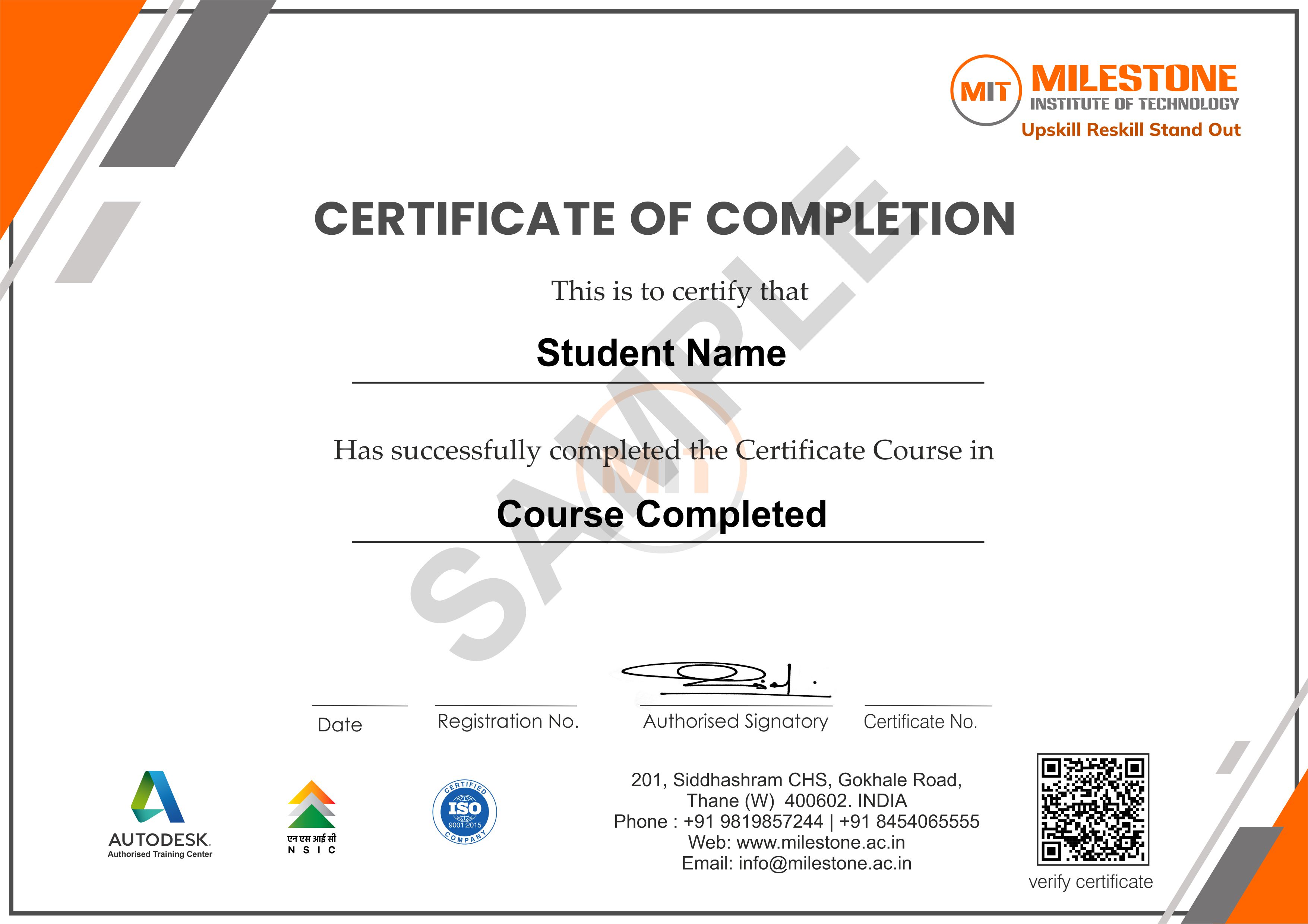
Capstone Projects In
This Coursework
Our projects are directly aligned with the coursework, ensuring practical application of what you learn. This hands-on approach deepens your understanding and prepares you for real-world challenges.
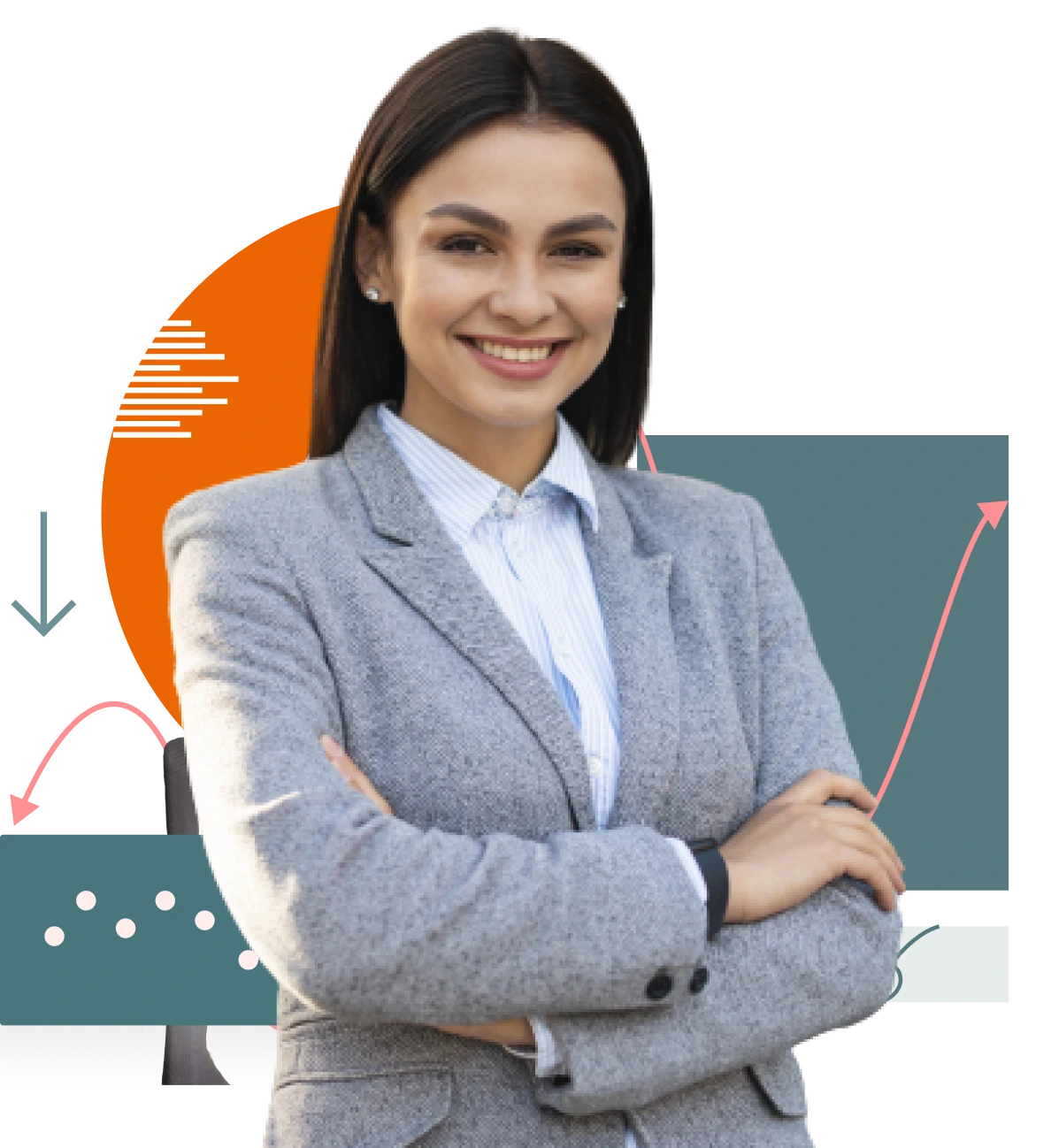
Get in touch today
Frequently Asked Questions
Find answers to all your questions about our diverse course categories. Discover the range of subjects we offer, and learn how to choose the right courses to match your interests and career goals. Let us guide you in navigating our extensive catalog to find the perfect fit for your educational journey.
-
Who can enroll for this course?
Any person with ME, MTech, BE, BTech, BCA, MCA, Msc, Bsc in Computer Science, Bsc IT, Msc IT, Diploma, IT, AI, ML can register for this course.
-
What will be the mode of delivery?
We offer 3 delivery models
1) Classroom
2) Live Online
3) Recorded lectures
Kindly contact us with your requirements. -
Will I receive a certificate after completion of this course?
Yes, you will be getting a certificate from Milestone Institute of Technology.
-
Does this course align with Industry requirements?
Yes, at MIT we ensure our syllabus and exercises are up to date as per industry requirements. We have used industry examples wherever possible in the course material. Additionally, you can also register your interest in Industry internships opportunities with our placement department.
to a whole new level?